API Overview
Overview of Parcl Labs offering and our roadmap
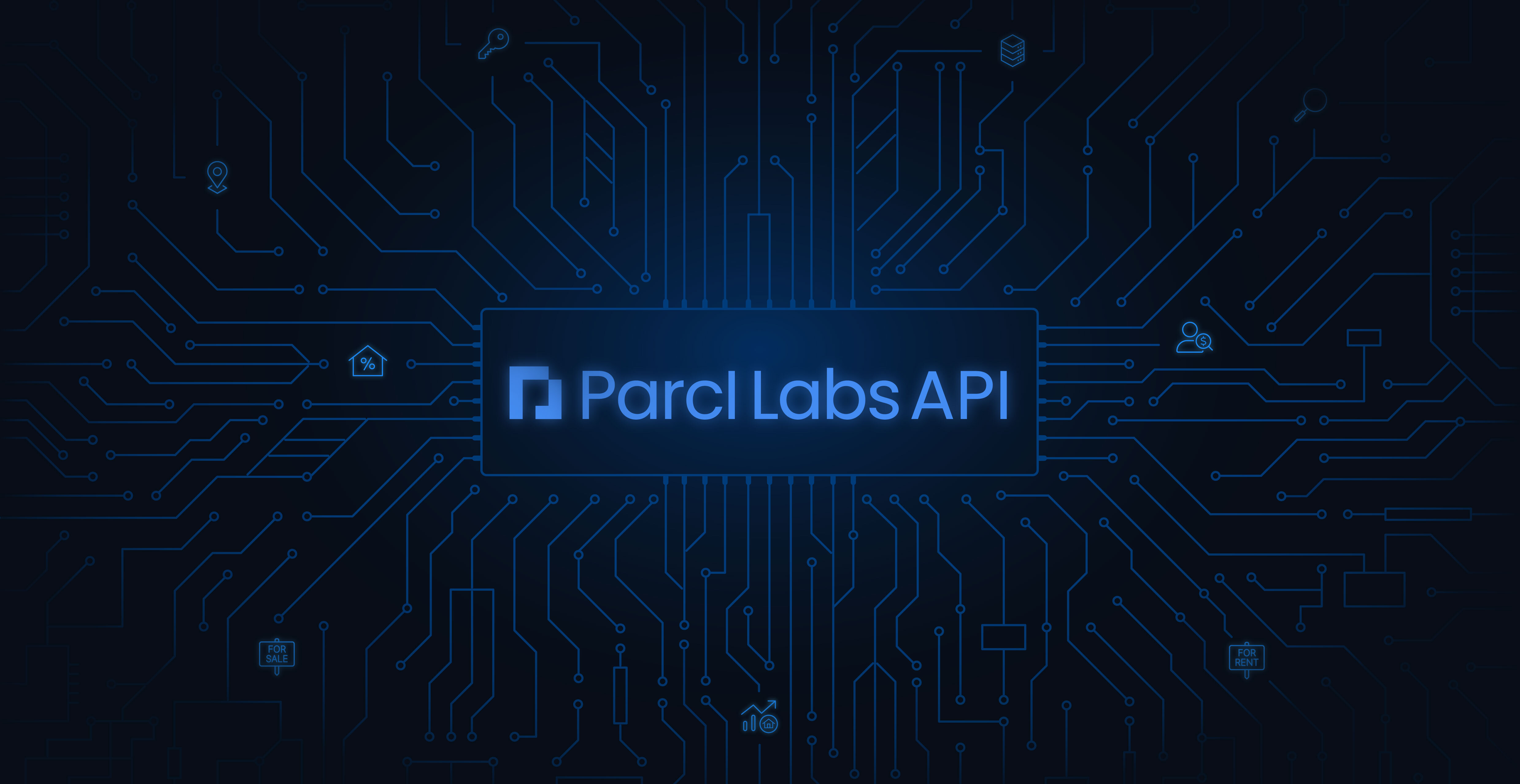
Welcome to the Parcl Labs API!
🔑 Need an API key? Start for free today.
🚀 Ready to build? Jump straight into the code with our Quickstart, API Reference, and SDK.
API Overview
The Parcl Labs API delivers real-time U.S. housing market insights, providing data on prices, supply, sales, listings, rentals, investor activity, and new construction at both market and unit levels.
The most complete picture of US residential real estate
Category | Coverage |
---|---|
Property Types | 🏘️ All Residential Assets: ✅ Single Family ✅ Townhouses ✅ Condos ✅ Other |
Markets | 🇺🇸 Complete National Coverage, 70k+ Unique Markets at Any Level of Granularity: ✅ Regions ✅ States ✅ Metros ✅ Cities ✅ Counties ✅ Towns ✅ Zips ✅ Census Places |
Housing Events | 🔄 The Full Property Lifecycle: ✅ Sales ✅ For Sale Listings ✅ Rentals |
Available API Endpoint Categories
- Market Metrics: Data on overall housing stock, supply, and price trends across sales, listings, and rentals. Learn more.
- For Sale Market Metrics: Data on the for sale listing market, including real-time supply. Learn more.
- Rental Market Metrics: Data on the rental market, including real-time supply, rental unit concentration, and yields. Learn more.
- Investor Metrics: Analytics on overall investor ownership and transaction activities. Learn more.
- Portfolio Metrics: Analytics on investor ownership and transaction activity for single-family homes in markets, segmented by the unit size of the investor's portfolio. Learn more.
- New Construction Metrics: Data on supply, demand, and price trends in the new home market. Learn more.
- Price Feed: Real-time Parcl Labs Price Feed data. Learn more.
- Property: Unit-level data integrating property attributes with sales, listing, and rental events. Learn more.
Within each category, we've prioritized providing data points that are either:
- Foundational supply and price data as building blocks for your own analysis.
- High-value, advanced metrics unique to our platform.
And we're just getting started...
API Roadmap
We’ve partnered with top teams in real estate, finance, and academia to identify the housing data that moves the needle for decision-making. These partnerships have directly shaped what’s available today and our roadmap.
Our API is constantly evolving. We have an aggressive roadmap and ship new features weekly. Our developer community gets first access. Check out our Changelog to see recent updates, including:
- Real-time price feeds
- New construction metrics
- Property data
- True owner entities (e.g., Invitation Homes)
Some roadmap items we're excited about:
- Expanded true owner entities: SFR operators, homebuilders, iBuyers, banks, etc.
- Advanced housing event tracking (e.g., portfolio deals)
- Extended historical data coverage
- Global market support for Parcl Labs Price Feeds
- And much more.
We want to hear from you! If you have feedback on what you’d like to see, please post it in the community forum.
Updated 19 days ago